Overview
Hi! I am Marcus Phua and I am currently a Year 2 Computer Engineering undergraduate at the National University of Singapore (NUS). This is where I document my contributions to PlanMySem.
For our Software Engineering project, my team and I were tasked with enhancing a basic command line interface address book application.
We chose to morph it into a text-based (Command Line Interface) scheduling/calendar application called PlanMySem, which caters to NUS students and staff who prefer to use a desktop application for managing their schedule/calendar.
PlanMySem automatically creates a planner that is synchronised according to the NUS academic calendar for the current semester and enables easy creation, editing and deleting of items.
Special weeks such as recess week and reading week are taken into account within our unique recursion system.
Items can then be efficiently managed via the intuitive tagging system.
Note the following symbols and formatting used in this document:
This symbol indicates important information. |
Also take note:
|
A grey highlight (called a mark-up) indicates that this is a command that can be inputted into the command line and executed by the application. |
|
Blue text with grey highlight indicates a component, class, method or object in the architecture of the application. |
My role was to design and write the codes for the list
, find
, history
, undo
and redo
features.
The following sections illustrate these enhancements in more detail, as well as
the relevant sections I have added to the user and developer guides in relation to these enhancements.
Summary of contributions
This section shows a summary of my coding, documentation, and other helpful contributions to the team project.
-
Main Feature: I implemented the searching framework within the
Planner
. The searching framework comprises of 2 sections:-
List: I added the ability to search and list
Slots
using a keyword user input.-
What it does: The
list
command generates a list containing allSlots
whose name/tag directly matches the specified keyword (not case-sensitive). -
Justification: After the user has added
Slots
to their planner, they may want to be able to view information regarding aSlot
that they have added. If the user wants to find out the dates, start times, end times, venues and descriptions pertaining to a specificSlot
, they can use thelist
command to locate that specificSlot
and view all of its information in a list.
-
-
Find: I added the ability to search and find
Slots
using a keyword user input.-
What it does: The
find
command generates a list containing allSlots
whose name closely matches the specified keyword and displays them as a list. -
Justification: Unlike the
list
command, thefind
command will also consider searches that are similar to the desired name/tag. This is to account for a typo or an incomplete keyword, which can happen often while the user is typing. Additionally, the user may not remember the exact name he has added to the planner, and hence, thefind
command provides a higher chance for the user to locate their desiredSlot
.The find
command ends up generating a longer output list, which potentially contains manySlots
, which may not be optimal for the user to skim through. Thus, I provide users with the option to use thelist
command to specifically pinpoint their desiredSlot
to list. -
Highlights: It was challenging to provide an optimal string matching algorithm that accurately weighs the closeness of strings. An in-depth analysis of design alternatives was necessary to determine the kind of string matching algorithm to implement in order to improve search accuracy.
-
Credits: The string matching logic was largely dependent on the Levenshtein Distance algorithm.
-
-
-
Minor Feature: I added the ability to undo/redo previous commands and view the history of commands entered.
-
What it does: The
undo
command allows the user to undo a previousadd
/edit
/delete
command. The user may reverse this command with theredo
command. -
Justification: In the event that users have made a mistake or changed their minds about executing a command, the
undo
command enables them to revert to a version immediately before the mistaken command was executed. If they change their minds again and decide to execute the command after all, then theredo
command enables them to do so easily. -
Highlights: As our group chose to morph the product from AddressBook 3, we did not have a History framework in place. I took up the responsibility to adapt the History Framework provided in AddressBook 4 in order to cater to our implementation of PlanMySem.
-
Credits: AddressBook 4 for providing the History framework.
-
To get a sense of how all these features function together, the activity diagram below shows how the search workflow for a Slot
in PlanMySem looks like:
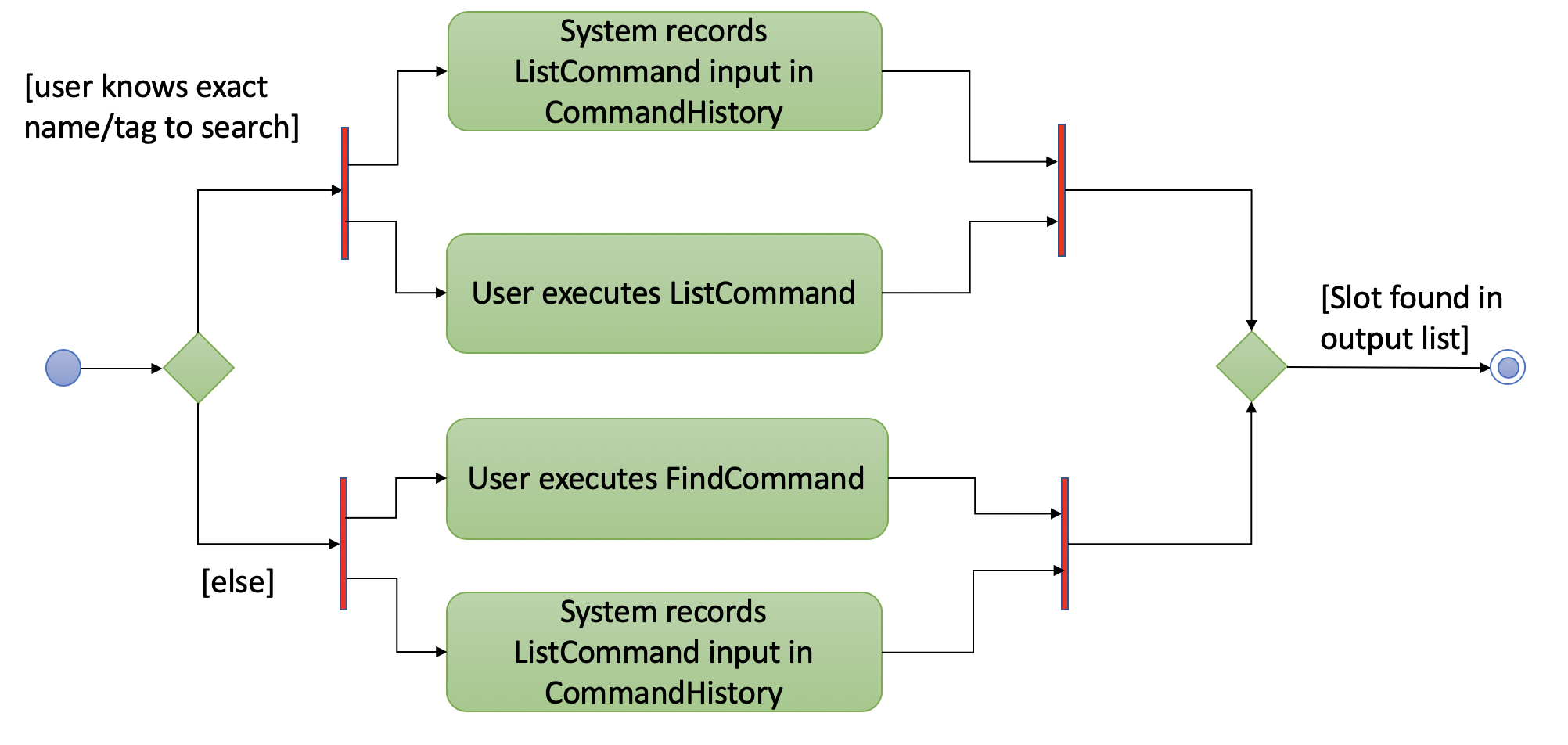
Essentially, if the user knows the exact keyword to use to locate his/her desired Slot
, he would use the list
command.
Otherwise, the find
command is recommended for use. As a parallel process, CommandHistory
records the user input and saves it to Planner’s
history.
-
Code contributed: [RepoSense] [Pull Requests]
-
Other contributions:
-
Project management:
-
I managed releases from version 1.1 to 1.4, which are all of the releases on GitHub.
-
-
Enhancement to existing features:
-
I wrote additional tests for existing features to increase coverage.
-
-
Documentation:
-
Community:
-
Tools:
-
I completed the initial set up of the developer team and organisation repository on GitHub.
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. I have added the descriptions of all features that I have implemented. I have also provided clear instructions for users to use my implemented features. |
Here are the links to my contributed sections in the User Guide:
Below is an example of my addition to the User Guide for the find
feature.
Finding Slots: find
/ f
Find all slots whose name closely matches the specified keyword and displays them as a list. (Case-sensitive)
Format: find n/KEYWORD
Keywords are case sensitive! (e.g. CS2113T is not the same as cs2113t) |
The find command will return the closest matching Slot which contains the specified keyword. The name/tag MUST
contain the specified keyword in order for a match to occur.
|
Use short keywords (e.g CS) instead of long keywords to increase the chances of finding your desired slot. E.g. Let’s say you are finding a Slot named Golf.find n/Go will detect the slot, while find n/Golfs will fail to detect the slot. (Golf does not contain Golfs) |
Example:
-
find n/CS
Find all slots whose name closely matchesCS
(eg. CS2101, CS2113T, SOCSMeet) -
find t/2113T
Find all slots that contain tags that closely matches2113T
.
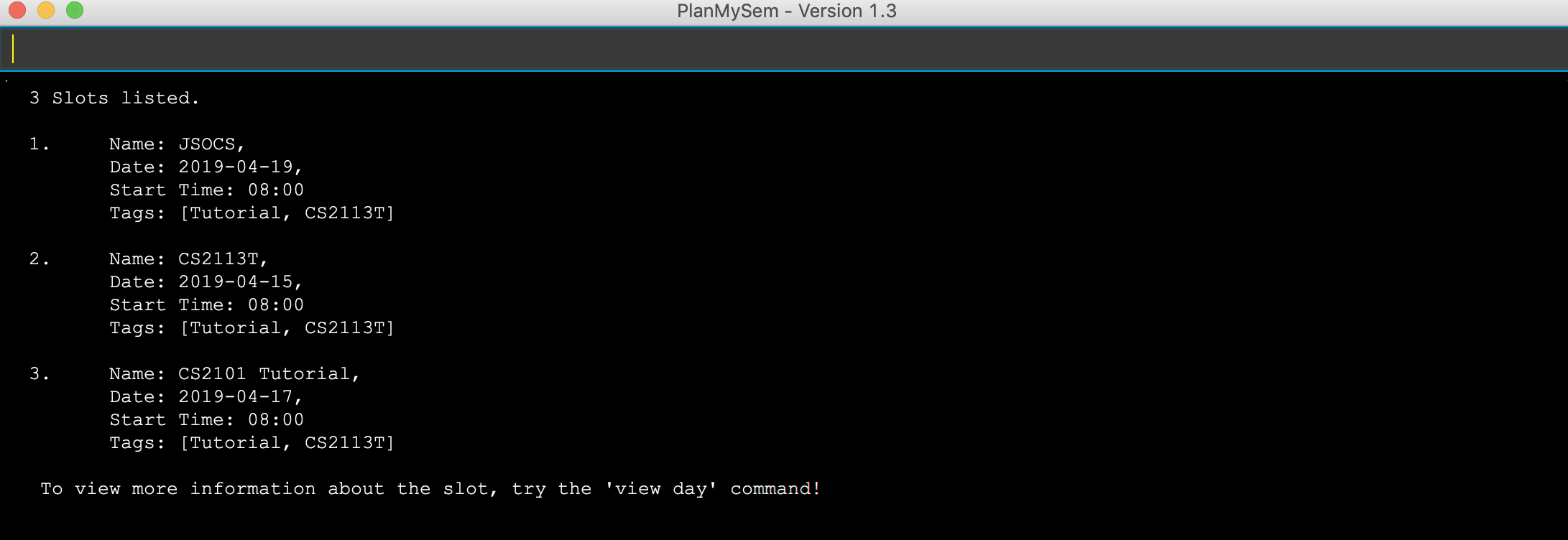
find n/CS
Explanation: As seen from the figure above, the output list of slots are ranked according to their degree of similarity.
All of the Slots
listed contain the keyword 'CS'.
Since 'JSOCS' has less characters, it is considered closest to the keyword 'CS', which has only 2 characters.
Remember the tip above when you are finding your Slots
.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. I have explained the logic behind the features that were tasked to me.
I have also elaborated on my design considerations in order to justify my implementation style. In addition, I was tasked with collating and polishing the User Stories which were generated during the ideation phase of the project. |
Here are the links to my contributed sections in the Developer Guide:
-
List Command:
list
-
Find Command:
find
-
Undo & Redo Command:
undo
&redo
-
User Stories: User Stories
Below is an example of my addition to the Developer Guide for the find
feature.
Find feature
Current Implementation
The find function supports searching using a single keyword.
The name/tag of the Slot MUST contain the specified keyword in order for a match to occur. Completely different keywords
do not constitute a match. |
The matching Slots
are then weighted based on their name/tag’s Levenshtein Distance from the keyword.
A low Levenshtein Distance is attributed to a high level of similarity between the name/tag and the keyword.
(A value of 0 constitutes an exact match.) The maximum Levenshtein Distance set in PlanMySem is 20. |
The weighted Slots
are inserted into a PriorityQueue
and the closest matching Slots
will be polled into the output list.
Upon executing the find
command with valid parameters,
a sequence of events is executed. The sequence of events illustrated in the Sequence Diagram below will be in reference to the execution
of a find n/keyword
command. The sequence of events are as follows:
-
Upon calling the
execute
method of theLogic
component, theLogic
component would then parse thefind n/keyword
command. -
LogicManager
then invokes theparseCommand
function ofParserManager
. -
ParserManager
in turn invokes theparse
function of the appropriate parser for thefind
command which in this case, isFindCommandParser
. -
After parsing is done,
FindCommandParser
would instantiate theFindCommand
object which would be returned to theLogicManager
. -
LogicManager
is then able to invoke theexecute
function of the returnedFindCommand
object. -
The command execution will call the
getDays
method of theFindCommand
object which retrieves data from theModel
component (i.e. retrieving data from the currentSemester
). -
FindCommand
will execute thegetDiscoveredNames
method to find the closely matchingSlots
with names containing 'keyword'. -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back toUi
. -
In addition, the
CommandResult
object can also instruct theUi
to display results, such as displaying help to the user.
To give a graphical summary of the above process, a Sequence Diagram detailing the execution of the find n/keyword
command is provided below.
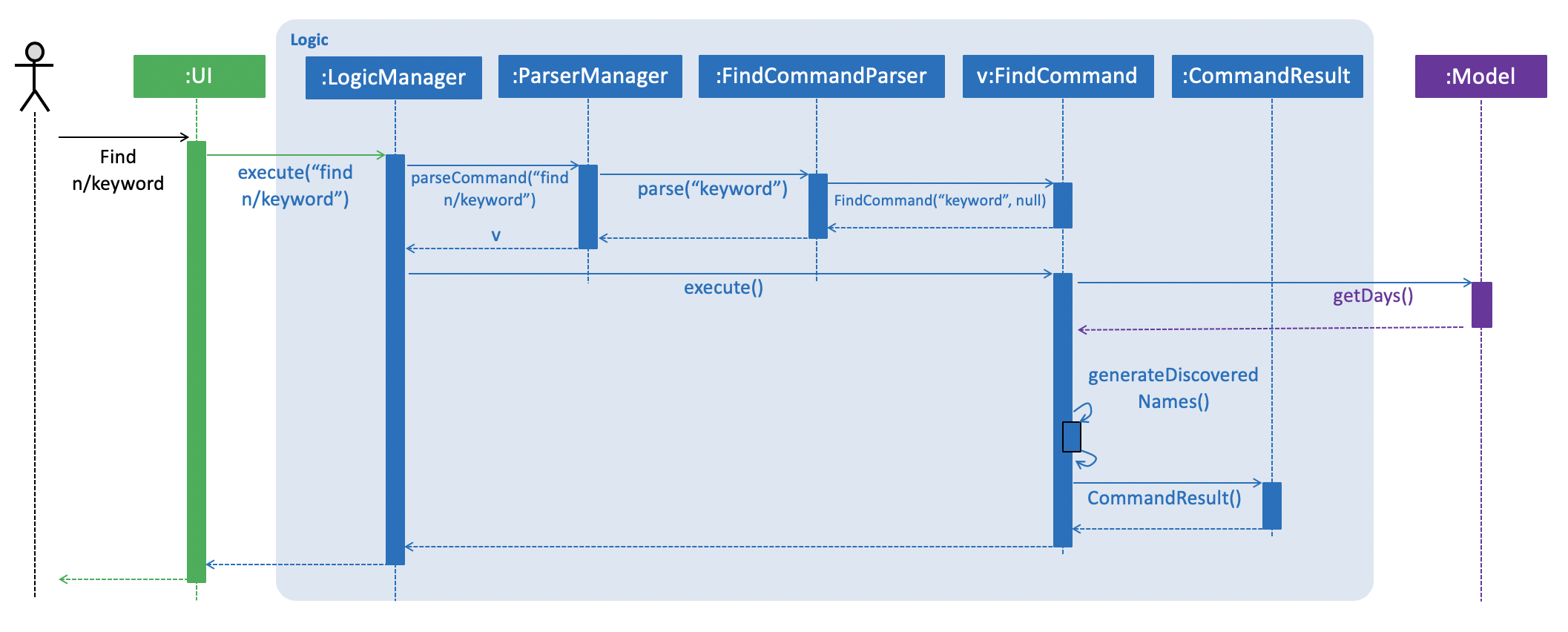
find n/keyword
CommandThe Communication Diagram below summarises the data links between the various objects in the find
command.
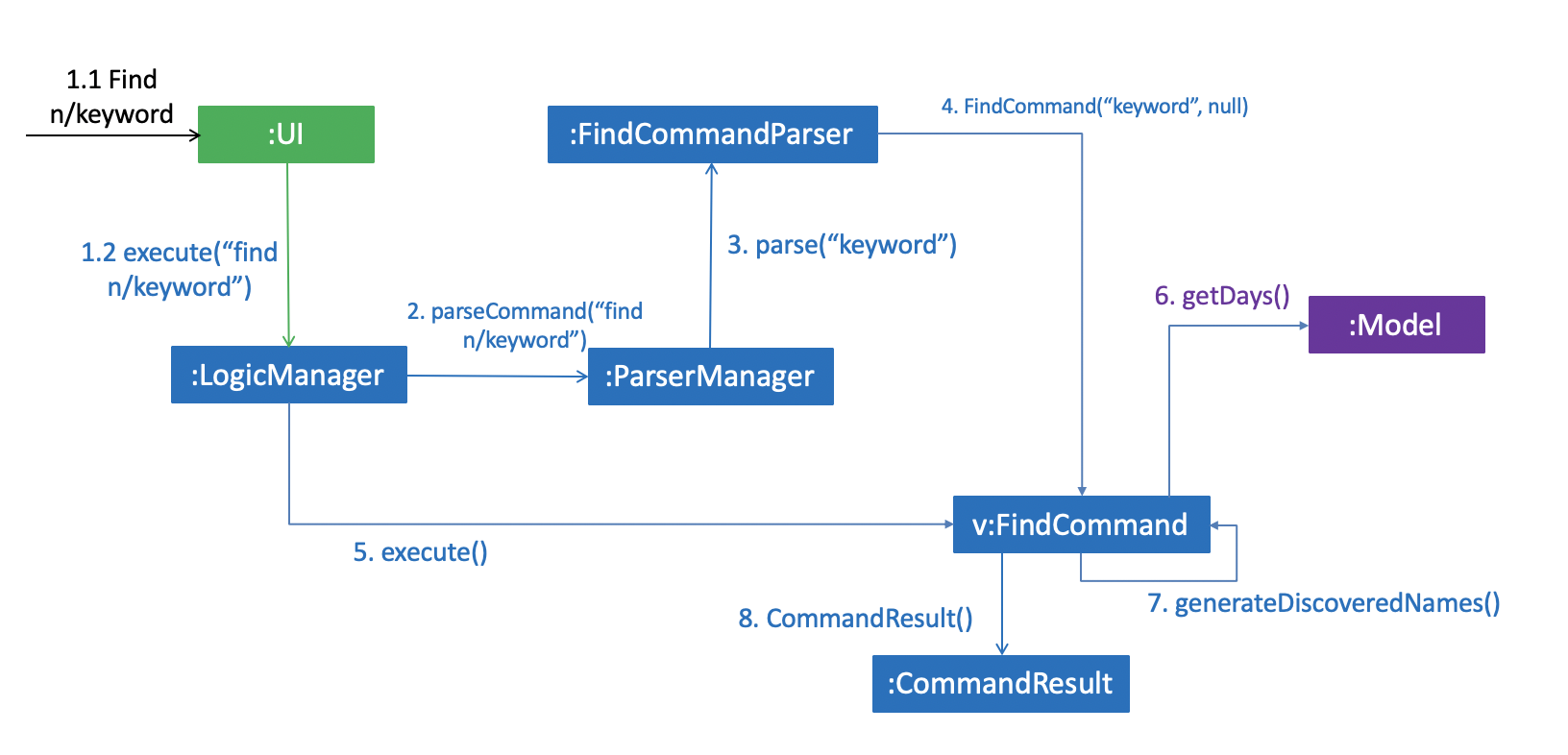
find n/keyword
Command
Design Considerations
Aspect: What constitutes a positive search result in find
command
No. |
Alternatives |
Pros |
Cons |
Past Implementation 1 |
Positive search result by strictly matching the entered keyword |
Easy to implement. |
Search must be exact, typos or an incomplete keyword will yield incorrect results. Nothing different from |
Past Implementation 2 |
Positive search result as long as name/tag contains the keyword. |
Searches will detect names/tags similar to the keyword. |
Output list will be longer. May become excessively long if short keyword is provided. |
Current Implementation |
Store the search results in a |
Searches are ordered by a degree of similarity, instead of the random order of names/tags in Past Implementation 2. |
Adds complexities in finding and searching. |
Glossary
- Levenshtein Distance
-
The Levenshtein distance is a string metric for measuring difference between two sequences.
Informally, the Levenshtein distance between two words is the minimum number of single-character edits (i.e. insertions, deletions or substitutions) required to change one word into the other.